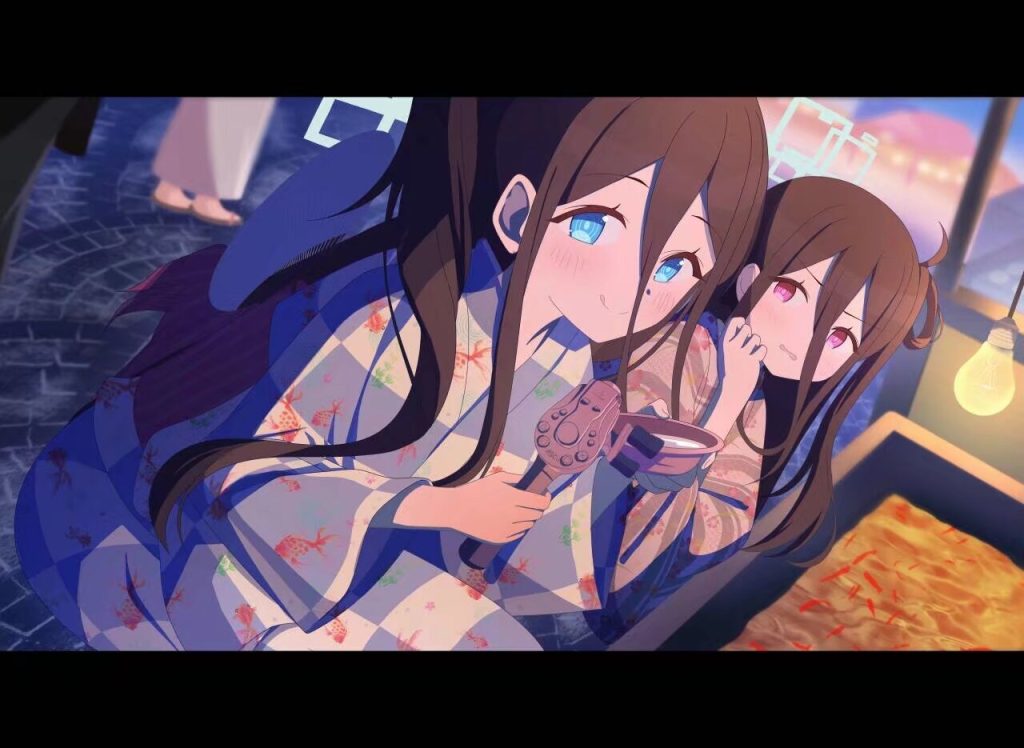
去停用词,这个概念听起来可能有点专业,但其实意思很简单。想象一下,你写了一篇文章或者一段话,里面有很多“的”、“了”、“在”、“是”这样的词,它们对于句子的结构很重要,但在很多情况下,对于理解文章的主要意思或者进行文本分析时,这些词并不提供太多有用的信息。这些频繁出现但又对内容理解帮助不大的词,我们就叫它们“停用词”。
“去停用词”,顾名思义,就是把这些不太重要的词从文本中去掉的过程。这样做的好处是,可以让文本变得更加精简,同时让重要的、有实际意义的词汇更加突出,方便我们或者机器更好地理解和分析文本的主要内容。
举个例子,如果你有一段话:“我今天在公园里看到了一个红色的气球。” 去停用词后,可能会变成:“我今天公园看到红色气球。” 虽然句子变得不那么通顺了,但对于某些文本分析任务来说,这样处理后的文本可能更容易处理,因为去掉了那些对分析意义不大的词汇。
所以,简单来说,去停用词就像是给文本“瘦身”,去掉那些虽然常见但对理解内容帮助不大的词,让文本的重点更加突出。
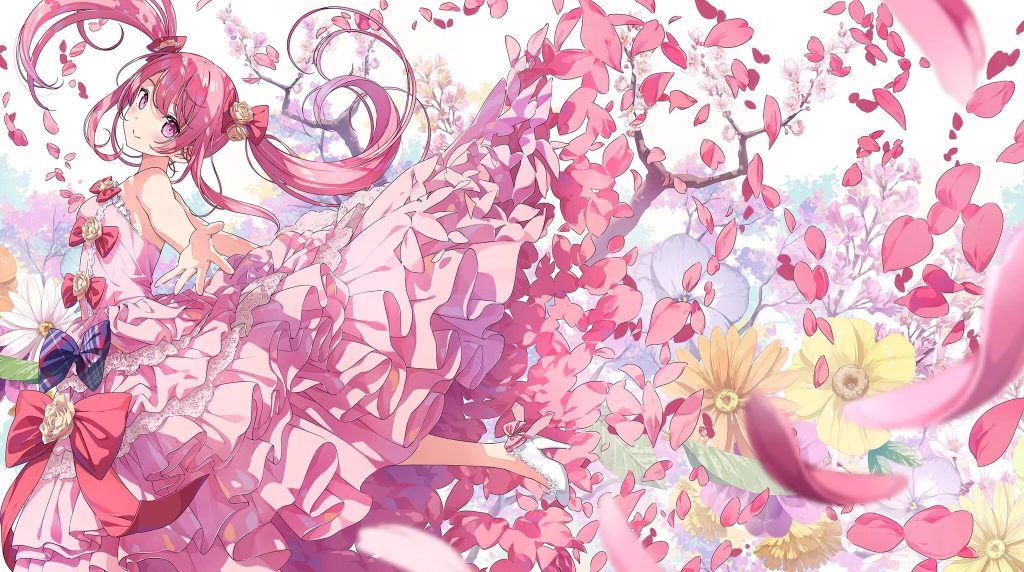
使用NLTK去停用词
import nltk
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
# 下载必要的NLTK资源(只需运行一次)
nltk.download('punkt')
nltk.download('stopwords')
# 示例文本
text = "This is a sample sentence, showing off the stop words filtration."
# 步骤1:分词(将文本拆分成单词)
words = word_tokenize(text)
# 步骤2:加载英文停用词列表
stop_words = set(stopwords.words('english'))
# 步骤3:去停用词
filtered_words = [word for word in words if word.lower() not in stop_words]
# 打印结果
print(filtered_words)
逐行分析:
import nltk
:导入NLTK库。from nltk.corpus import stopwords
:从NLTK库中导入停用词模块。from nltk.tokenize import word_tokenize
:从NLTK库中导入分词函数。nltk.download('punkt')
和nltk.download('stopwords')
:下载分词器和停用词资源(这是第一次使用时需要做的)。text = "This is a sample sentence, showing off the stop words filtration."
:定义示例文本。words = word_tokenize(text)
:使用word_tokenize
函数将文本拆分成单词列表。stop_words = set(stopwords.words('english'))
:加载英文停用词列表,并将其转换为一个集合(set),以便快速查找。filtered_words = [word for word in words if word.lower() not in stop_words]
:使用列表推导式去停用词。对于words
列表中的每个单词,如果它(转换为小写后)不在stop_words
集合中,则将其添加到filtered_words
列表中。print(filtered_words)
:打印去停用词后的单词列表。
使用SpaCy去停用词
import spacy
# 加载英文模型
nlp = spacy.load("en_core_web_sm")
# 示例文本
text = "This is a sample sentence, showing off the stop words filtration."
# 步骤1:使用SpaCy处理文本
doc = nlp(text)
# 步骤2:去停用词
filtered_words = [token.text for token in doc if not token.is_stop]
# 打印结果
print(filtered_words)
逐行分析:
import spacy
:导入SpaCy库。nlp = spacy.load("en_core_web_sm")
:加载英文模型(这里使用的是小型模型en_core_web_sm
)。text = "This is a sample sentence, showing off the stop words filtration."
:定义示例文本。doc = nlp(text)
:使用SpaCy模型处理文本,生成一个Doc
对象。filtered_words = [token.text for token in doc if not token.is_stop]
:使用列表推导式去停用词。对于doc
中的每个token
(即单词或标点符号等),如果它不是停用词(token.is_stop
为False
),则将其文本(token.text
)添加到filtered_words
列表中。print(filtered_words)
:打印去停用词后的单词列表。
(仅供参考)
Comments 1 条评论
太TM牛逼了